Hey everyone. The work that has been completed since the last update has been all about creating the most basic components of the game needed to begin working on actual gameplay. These components revolved around giving the player a gun that they can shoot at an enemy. As part of this process, I added in a couple of external content into the project. The first is a Sci-fi weapons pack by ByteSumPi LTD (available here) , then I added in the Sci-fi Weapon sound FX pack by AHPRODUCTION (available here) and lastly, I download an alien model from mixamo.
Creating the Player's Weapon
The first thing I worked on was attaching a weapon to the player. When deciding on how to go about this, I kept in mind that I needed to make it more logical and simpler to use than my pervious first-person weapon attempt that I used in blueprints. My initial idea for doing this was to have the weapon be a custom actor component of the player. This would also work well as it would make attaching weapons to any entity much easier and without the need to create duplicate classes. However, after spending some time working on this, the problems with this method started to show. The main problem is that actor components do not have a transform (at least, they cannot easily have one from what I can figure out). This means that an object of the weapon component class cannot be seen in the world, especially any meshes that are included in the component. I did manage to setup a system that gets the skeletal mesh variable in the weapon component class and assigns it to a skeletal mesh component on the player. This way of creating the player weapon did work, but I felt that I could do better and that it wasn’t the most logical way of achieving this.

So I settled on having the player’s weapons as just normal actors. This means that the weapon not only has a transform, making it much easier to work with, but it has a physical existence in the world making it much easier to gameplay such as dropping weapons (although I don’t play on necessarily having weapons be dropped). The only decision on this that needed to be made now was how to handle the player having multiple weapons to switch between. In the end I decided to have all the weapons that the player is using in the level spawn initially, before setting only the weapon the player is currently using to be functional and visible.


This effectively creates a system of object pooling, which makes the swapping of weapons quite efficient as there is no creating and destroying of skeletal meshes involved in switching guns. When the player switches guns, the old gun is just disabled and made invisible while the next gun is enabled and made visible, making a smooth transition between switching gun. The weapons were attached to the player camera (with an offset) so that they move around with the camera whenever the player looked around (as the camera’s rotation is adjusted when the player uses the mouse, not the player’s rotation).

Firing the Weapon
Once the player was able to hold a gun, the next step was to have the player be able to fire said gun. This ended up being more of an issue than I anticipated. Initially I created a transform variable that would be used to spawn the bullet. The problem occurred as this location would not easily update when the player moved and looked around. Eventually I decided to have an empty mesh component that would sit at the location that the bullets would spawn.

When the bullet is created, the location and rotation for the bullet would be taken from the actor component. This means that no matter where the gun is and what origination it has, the bullet will always fire forward. However, much later on I discovered a better way of doing this. Instead of having an empty mesh component to spawn the bullet from, I could just use a socket from the skeletal mesh to spawn the bullets from. Thankfully the weapon mesh pack that I am using does have sockets for the location for spawning bullets already added (although it wouldn’t have been difficult to add in a socket myself).

The next problem that occurred when having the bullets spawn was to have them act like a projectile. Initially on the bullet class I attached a projectile component to the bullet and set the max and initial speeds to a variable from the gun that spawned it. However, when I began testing the gun, the bullets would just fall out of the gun with no velocity. The reason this was happening confused me for ages and caused a lot of frustration. Eventually I needed to more than just set the speed variables in the projectile component. What I needed to do was to also set the velocity variable of the bullet. The velocity of the bullet needed to be set equal to the forward vector of the bullet multiplied by the initial velocity. Once this was all wrapped up in a function, the bullets could be fire from the gun.

However, while the player is now able to fire a gun, there were still some aspects that needed to be added to create a sensible firing system. The first of these was to add in ammo and reloading. This process was relatively simple. All it does is subtract the ammo the player has used from spare ammo (and adds it to the player’s gun). If the spare ammo drops below 0, the amount needed to get back to 0 is subtracted from the player’s gun ammo. This is a system that I have used before and it is pretty robust way of handling gun reloading.

The other aspect that needed to added in was fire rates and different firing systems for automatic and semi-automatic weapons. The firing system that I had already made was effectively an semi-automatic firing system anyway, so the only addition that needed to be made was to control the rate the player can fire. This was done by using a bool that gets reset after a time determined by the guns firing rate.

The problems came when trying to implement the automatic firing system. The first issue is that there is no what to check from within the engine if the player is holding the mouse button, so a separate bool had to be added that gets set to true when the mouse is clicked and set to false when released.
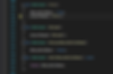
The next (and major) problem was getting the fire function to be called repeatedly but only when the gun can fire while the player is holding down the mouse. The fire function is only called when the input of LMB is activated, so it can only be called once per click. Trying to call it repeatedly any other way would often cause infinite loops as it would call the fire function too many times. The solution that I ended up going with was adding a check in the ResetFire function to check if the gun was an automatic gun. If this was true, then this function would call the fire function again (which does check if the player is holding down the fire button). This solution means that the fire function can only be called when the weapon is able to be fired.


Creating an Enemy to shoot
The final piece of the puzzle was to setup the most basic enemy functionality. All this really involved for the moment was to have an enemy class that stored a bunch of necessary variables and setup a skeletal mesh, as well as allowing the enemy to take damage from the player’s bullet.


This last part was the part that caused the most issues. This was mainly due to figuring out the correct signature that is needed on a function that is called on an overlap event. This problem was made harder to decipher as the error thrown on the “Add Dynamic” call only started that there was no overload function for the current arguments. While it was technically correct, it gave no indication that the function itself was the problem which mean a lot of time trying to solve the problem. However, in the end I did manage to get it all working.

Changes to the Firing system
It was at this point I decided to take a look back at the firing of the gun. I added in a simple crosshair to the screen and noticed that the guns do not fire towards the middle of the screen.
This got me thinking about exactly how I wanted the firing of the guns to work. If I wanted to have a crosshair (like most FPS games have), then I needed to have the guns firing towards the actual crosshair. My first attempt at this was to have the gun rotate towards a point along a vector from the centre of the player’s camera. This point would either be an object that the player is firing at or the point that represents the guns range (whichever would be closer). However, this attempt ended up being disastrous. The unreal function that rotates an actor towards a point is a blueprint callable only (meaning it can’t be used in c++ code) and any alternative ways at trying to rotate the gun ended up causing all sorts of weirdness.

I then tried to rotate the bullets after they spawned. This would effectively redirect the bullets towards the centre of the screen. However, this didn’t seem to have any effect.

It was at this point I pondered with even having the idea of a crosshair in the first place but decided that this would be something that would be better decided on from playtesting. So the simple solution for now was to just realign the guns rotation manually so that they fired towards the crosshair.

What's Coming Next
Now that the absolute core components have been created, I can begin work on creating the game’s gameplay. The most important part of this will be to setup a level that resembles the types of levels that the player will play in. This will also involve setting up the goods that the player has to defend and creating a navigation system for the enemies to use (this will either use the unreal engine navigation system or I might create my own. This is yet to be decided) so that they navigate around the map towards the goods. Once these important aspects are created, I then plan to add in some animations to the enemies and create the basic UI. So until the next devlog, goodbye.